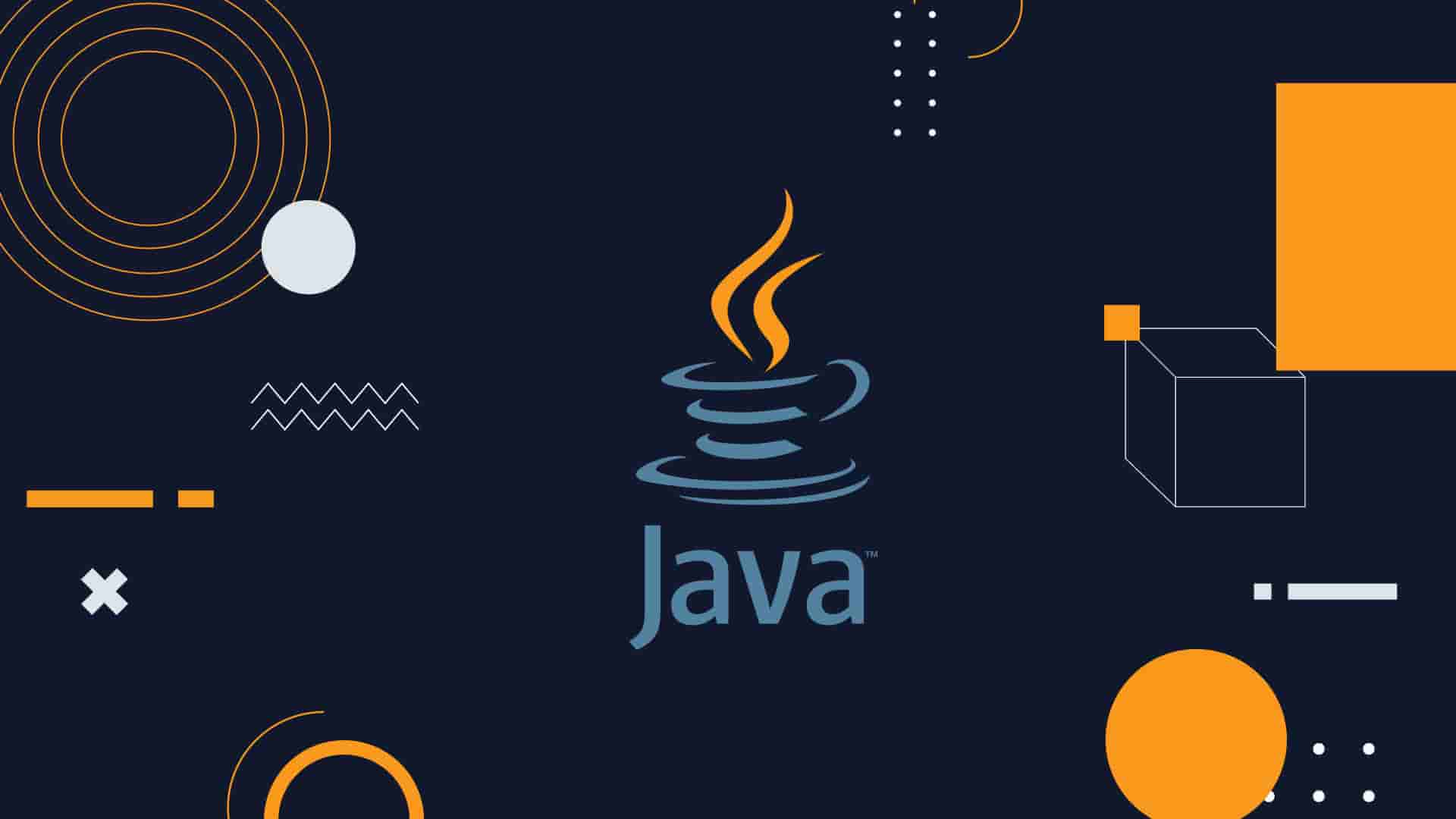
Formatting Dates in Java
Using the SimpleDateFormat classes to format date strings
Written by: Alex Root-Roatch | Tuesday, August 20, 2024
A Date with Java
Getting the current date and time in Java is easy with new Date()
, but the format of the date it returns is often
something like "Tues Aug 20 2024 01:07 PM" (depending on the country and region settings of the system). This is often
less desirable compared to something like "08/20/2024 13:07."
With SimpleDateFormat,
we can define a specific format for how a date should be turned into a string and how a string
should be parsed into a date.
Define a Pattern
First, we need to define the pattern of how the date is formatted. This will be a String
that will later be given to
the SimpleDateFormat
constructor.
For example:
String pattern = "yyyy-MM-dd HH:mm:ss";
SimpleDateFormat simpleDateFormat = new SimpleDateFormat(pattern);
Now with a date format defined, we can create a new date object that is formatted with this pattern:
String myDate = simpleDateFormat.format(new Date());
The characters in the pattern are case-sensitive. Notice that months are denoted with capital "M" but minutes are lowercase. Similarly, capital "H" denotes 24 hour time with 0 being midnight, while lowercase "h" is 12 hour time.
The number of characters provide also matter. For example, three capital "M's" will provide the first three letters of the month, but four will provide the full name of the month.
Pattern Syntax
I found this handy table in Digital Ocean's blog:
Letter for Pattern | Date or Time component | Examples |
---|---|---|
G | Era designator | AD |
y | Year | 2018 (yyyy), 18 (yy) |
M | Month in year | July (MMMM), Jul (MMM), 07 (MM) |
w | Results in week in year | 16 |
W | Results in week in month | 3 |
D | Gives the day count in the year | 266 |
d | Day of the month | 09 (dd), 9(d) |
F | Day of the week in month | 4 |
E | Day name in the week | Tuesday, Tue |
u | Day number of week where 1 represents Monday, 2 represents Tuesday and so on | 2 |
a | AM or PM marker | AM |
H | Hour in the day (0-23) | 12 |
k | Hour in the day (1-24) | 23 |
K | Hour in am/pm for 12 hour format (0-11) | 0 |
h | Hour in am/pm for 12 hour format (1-12) | 12 |
m | Minute in the hour | 59 |
s | Second in the minute | 35 |
S | Millisecond in the minute | 978 |
z | Timezone | Pacific Standard Time; PST; GMT-08:00 |
Z | Timezone offset in hours (RFC pattern) | -0800 |
X | Timezone offset in ISO format | -08; -0800; -08:00 |
Specifying Locale
We can provide a second argument to the SimpleDateFormat
constructor to specify the language and country of our date string:
String pattern = "yyyy-MM-dd HH:mm:ss";
SimpleDateFormat simpleDateFormat = new SimpleDateFormat(pattern, new Locale("es", "ES"));
This will return a date in Spanish with formatting specific to Spain. The first argument, "es", denotes the language, while the second denotes the country. For English in the United States, it would be new Locale("en", "US)
.
Locales are a combination of the ISO 639-1 language code and ISO 3166-1 country code.
Parsing
We can also turn strings into date objects with simpleDateFormat.parse()
. In addition to providing a string to be parsed, we still need to provide a pattern string so the method knows how to parse the string. For example:
String pattern = "yyyy-MM-dd HH:mm:ss";
SimpleDateFormat simpleDateFormat = new SimpleDateFormat(pattern);
Date date = simpleDateFormat.parse("2024-08-20 13:57:32");
This may look almost identical to the example before. The main difference is that the result is of type Date
instead of String
.